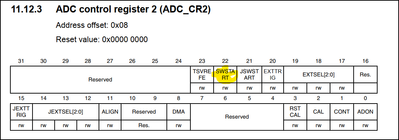
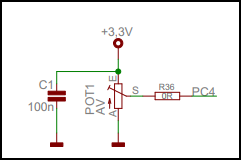
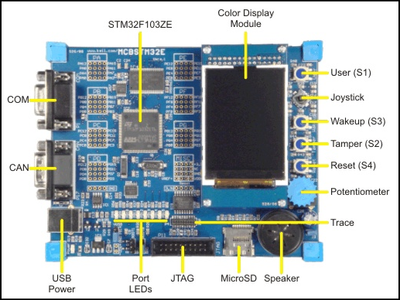
I'm learning STM32 with the board above and trying to program the ADC conversion.
However, when i start debug with keilC and observe the Adc value, nothing happen.
I check the ADC register and realized the SWSTART can not be set to start conversion process.
this is my source code:
#include "adc.h"
uint32_t Adc_value =0;
/*This code is applied for ADC12_IN14 GPIO:PC4*/
void ADC_Init_temp(void)
{
/************** STEPS TO FOLLOW *****************
1. Enable ADC and GPIO clock
2. Set the prescalar in the Clock configuration register (RCC_CFGR)
3. Set the Scan Mode and Resolution in the Control Register 1 (CR1)
4. Set the Continuous Conversion, EOC, and Data Alignment in Control Reg 2 (CR2)
5. Set the Sampling Time for the channels in ADC_SMPRx
6. Set the Regular channel sequence length in ADC_SQR1
7. Set the Respective GPIO PINs in the Analog Mode
************************************************/
/**/
/*1. Enable ADC and GPIO clock*/
RCC->APB2ENR |= (1<<4); /* Enable clock GPIOC*/
RCC->APB2ENR |= (1<<9); /*Enable clock ADC1*/
/*2. Set the prescalar in the Clock configuration register (RCC_CFGR)*/
RCC->CFGR |= (2<<14); /*PCLK2 divide by 6.... ADC_CLK = 72/6 = 12MHz */
/*3. Set the Scan Mode and Resolution in the Control Register 1 (CR1)*/
/*Scan mode must be set, if you are using more than 1 channel for the ADC.*/
ADC1->CR1 &= ~(15<<16); /*independen mode*/
/*4. Set the Continuous Conversion, DMA, and Data Alignment in Control Reg 2 (CR2)*/
ADC1->CR2 |= (1<<1); /*Enable Continuous conversion mode*/
ADC1->CR2 &= ~(1<<11); /*set right align*/
/*ADC1->CR2 |= (1<<8); Enable DMA*/
/*5. Set the Sampling Time for the channels in ADC_SMPRx*/
ADC1->SMPR1 &= ~(7<<12); /* Sampling time of 3 cycles for channel 11*/
/*6. Set the Regular channel in ADC_SQR1 and sequence in ADC_SQR3*/
ADC1->SQR1 &= ~(15<<20); /*0000: 1 conversion*/
ADC1->SQR3 |= (14<<0); /* channel 14 for sequence 1*/
/*7. Set the Respective GPIO PINs PC4 in the Analog Mode GPIOx_CRL*/
GPIOC->CRL &= ~(15<<16); /**/
}
void ADC_Enable(void)
{
uint32_t delay = 1000;
/*1. Enable ADC and to start conversion*/
ADC1->CR2 |=(1<<0); /*ADON enable*/
while (delay--);
}
void ADC_Start(void)
{
ADC1->SR &= 0; /* clear the status register */
ADC1->CR2 |= (1<<22); /*start coversion*/
}
int main(void)
{
ADC_Init_temp();
ADC_Enable();
ADC_Start() ;
while(1)
{
Adc_value = (ADC1->DR);
}
}